Animation_idle
1. Animation 창을 열고, Character를 누른후
2. Creat을 누르고 Character_idle이라는 animator를 만들고
3. Add property에서 Sprite Render에서 sprite선택
4. 처음이랑 끝이 같으므로 idle 끝냄

Animation_walk
1. Creat new clip에서 새로운 애니메이션 생성(creater_walk)
2. 이미지 드래그
3.
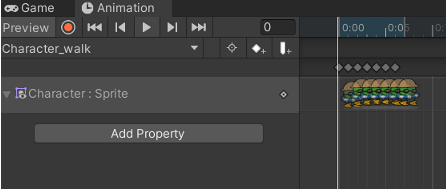
4. 애니메이터 창 클릭
5. 속도 0.2로 조절
idle -> walk transition
1. Has Exit Time: 체크해제
2. Parameters에서 bool로 iswalk 추가
3. conditions에서 iswalk true로
4.
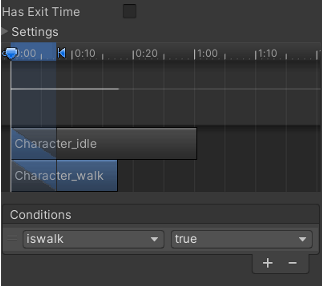
walk -> idel transition
1. Has Exit Time: 체크해제
2. conditions에서 iswalk false로
3.
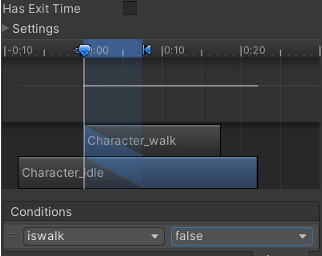
애니메이션 전환 스크립트 구성
Animator anim;
anim = GetComponent<Animator>();
anim.SetBool("iswalk", true);
anim.SetBool("iswalk", false);
• animator컴퍼넌트를 가져와서 setbool을 이용해서 마우스 클릭스 꺼지고, 켜지게 하기
오른쪽으로 이동할때 오른쪽보게 하기
Animator anim;
anim = GetComponent<Animator>();
anim.SetBool("iswalk", true);
anim.SetBool("iswalk", false);
//왼쪽으로 이동하는지 오른쪽으로 이동하는지
if(dir.x < 0)
{
transform.localScale= new Vector3(-1, 1, 1);
}
else
{
transform.localScale= new Vector3(1, 1, 1);
}
최종
PlayerControler
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlyayerControler : MonoBehaviour
{
public bool isJoyStick;
public float speed;
public GameObject joystick;
Animator anim;
private void Start()
{
anim = GetComponent<Animator>();
//카메라가 Player의 자식으로 들어감
Camera.main.transform.parent = transform;
//카메라가 자식들어갈때 위치지정
Camera.main.transform.localPosition = new Vector3(0, 0, -10);
}
private void Update()
{
Move();
}
void Move()
{
if (isJoyStick)
{
joystick.SetActive(true);
}
else
{
joystick.SetActive(false);
//클릭했는지 판단
if (Input.GetMouseButton(0))
{
//스크립트 상에서 나누기보다 곱하기가 계산속도가 빠름
//전체화면의 0.5를 곱하면 중앙이 되며, 이를 마우스 지점으로 지정하고, 정규화해주면 방향을 얻는 벡터가 됨
//클릭했는지 판단(마우스, 터치)
Vector3 dir = (Input.mousePosition - new Vector3(Screen.width * 0.5f, Screen.height * 0.5f)).normalized;
transform.position += dir * speed * Time.deltaTime;
//어떤값을 어떻게 바꿀것인가
anim.SetBool("iswalk", true);
//왼쪽으로 이동하는지 오른쪽으로 이동하는지
if(dir.x < 0)
{
transform.localScale= new Vector3(-1, 1, 1);
}
else
{
transform.localScale= new Vector3(1, 1, 1);
}
}
//클릭하지 않는다면
else
{
anim.SetBool("iswalk", false);
}
}
}
}
Joystick
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Joystick : MonoBehaviour
{
//1. Stick드래그 , 제한
//2. 드래그만큼 캐릭터 이동
// 다른 스크립트 불러오기
PlyayerControler plyayerControler_script;
// 스틱 누르면 호출
public RectTransform stick, background;
bool isDrag;
float limit;
Animator anim;
public void ClickStick()
{
isDrag = true;
}
void Start()
{
anim = GetComponent<Animator>();
plyayerControler_script = GetComponent<PlyayerControler>();
limit = background.rect.width * 0.5f;
}
// Update is called once per frame
void Update()
{ //드래그 하는 동안
if (isDrag)
{
Vector3 dir = (stick.position - background.position).normalized;
transform.position += dir * plyayerControler_script.speed * Time.deltaTime;
//마우스의 위치로부터 background중앙까지의 거리 계산
Vector2 vec = Input.mousePosition - background.position;
//vector2의 값을 제한(어떤값을, 얼마만큼 제한할지)
stick.localPosition = Vector2.ClampMagnitude(vec, limit);
anim.SetBool("iswalk", true);
//왼쪽으로 이동하는지 오른쪽으로 이동하는지
if (dir.x < 0)
{
transform.localScale = new Vector3(-1, 1, 1);
}
else
{
transform.localScale = new Vector3(1, 1, 1);
}
//드래그 끝나면
//마우스를 뗐을때는 부모의 위치에서 최초위치로 원복
if (Input.GetMouseButtonUp(0))
{
stick.localPosition = new Vector3(0, 0, 0);
isDrag = false;
anim.SetBool("iswalk", false);
}
}
}
}
댓글남기기